Go, why not ?
With more and more applications, Docker, InfluxDB, Kubernetes, etcd, Grafana, using Go as their foundation, it’s interesting to understand the reasoning behing it. In this article we’ll also share some pointers and cheatsheet to learn Go.
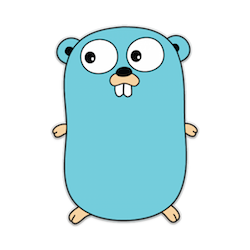
Introduction
Go was first introduced in 2007 at Google by Robert Griesemer, Rob Pike and Ken Thompson. The main objective was to solve their own issues. Google developpers were using C/C++, Java and Python, complexity was growing and it became harder to maintain a level of coherence between their different projects. All three creators shared their dislike of C++ complexity as a primary motivation for designing a new language.
The main design decisions were:
- statically typed
- be readable, small, simple, robust and light
- support networking and multiprocessing.
Installation
Go is currently available for Windows, MacOS, Linux and FreeBSD. Download and install Go from binaries available at Golang. As this time the current version is 1.7 which is a ~80Mb download but if you prefer you can install it from source.
Once installed add the following path to your shell environment variable
export PATH=$PATH:/usr/local/go/bin
Setup a workspace directory and export it as well as the bin
directory.
mkdir ~/in/code/go
export GOPATH=~/in/code/go
export $PATH:$GOPATH/bin
$GOPATH
directory will contain all the go code in the machine.
You can also create a directory for your own stuff
mkdir -p $GOPATH/src/github.com/user
just replace user
by your github username.
Pros
Go, also written Golang offers the following benefits:
- Compiled
- Statically linked native binaries without external dependencies
- Clean syntax
- Simple Type system
- Concurrency primitives
- Rich standard library
- Great tools
Go tour
An online guided tour is available just by typing:
go tool tour
You can also run everything locally like this
go get github.com/dupoxy/go-tour-fr/gotour
cd $GOPATH/bin
./gotour
Embedded Documentation
To serve the documentation locally Just type
godoc -http=:6060 -play -index
Go Tooling
- go help - more information about a command.
- go doc - documentation for any package
- go get - fetch dependencies online
- go fmt - format your source code automatically
- go test - runs all Test* functions in *_test.go
- go build - compile packages and dependencies
- go run - rapid script like prototyping
Additional Go commands
- go version - print Go version
- go list ./… - lists the packages named by the import paths, start in the current directory (“./”) and find all packages below that directory (“…”)
- go install - installs the latest copy of the package into the pkg directory
- go clean - removes object files from package source directories
- go tool - runs the go tool command identified by the arguments
- go vet - run go tool vet on packages which reports suspicious constructs.
Packages
Store your packages in a directory which match its source location
$GOPATH/src/github.com/yourname/ask
Corresponding Import Path is
import "github.com/yourname/ask"
Package names should be all lowercase
Conclusion
Go is easier to install, test and adopt. Its KISS (Keep it simple, stupid) culture allows to broaden the community.
go build
embed everything you need, no more install required in order to run what you need, having a single binary to distribute to your server is a great advantages (apart from libc !!!)
Go combines the development efficiency of interpreted or dynamic languages with the security of static languages.
Links
- awesomo - big list of interesting open source go projects
- Awesome Go - A curated list of awesome Go frameworks, libraries and software
- Go Wiki
- GoBooks
- Gopm Registry
Documentations
Intesting projects written in Go
- Beego - An open source framework to build and develop your applications in the Go way
- Caddy - Alternative web server that is easy to configure and use
- Minio - Object storage server, S3 compatible, built for cloud application developers and devops.
- Gogs - Go Git Service, a painless self-hosted Git service